In this Article, I will share code about creating a path travelling game in C++ using Graphics. I have used Dev C++ and Code Blocks compiler for testing this code. If you want to know how to setup code blocks for graphics watch this video
Description:
In this code,
- First of all I have printed Instructions on graphic screen about How to play the game. outtextxy function is used to print text on graphic window.
- After that I have created the path using line function.
- After that I have defined conditions where Lines are, If user stays in between those lined boundries, and keep pressing the buttons A(up), dot . (down), L(left), Z(right). The Circle on the screen keeps moving in that specific direction.
- If the User reaches center of the path, It wins, If it touches any line before reaching that, It loses.
- Clock function is used to record how much time user takes to play the game, and it displays the time on console at the end.
Code
#include<iostream>
#include<graphics.h>
#include<conio.h>
#include<stdlib.h>
#include<ctime>
using namespace std;
int main()
{
clock_t st;
int gdriver=DETECT,gmode;
initgraph(&gdriver,&gmode,"c:\\tc\\bgi");
cleardevice();
int x=125,y=125,key;
//print this text on screen
outtextxy(100,0,"PRESS ANY KEY TO SOLVE THIS BHOLBHALAYA");
outtextxy(100,20,"Z to move right");
outtextxy(100,40,"L to move left");
outtextxy(100,60,". to move down");
outtextxy(100,80,"A to move up");
outtextxy(10,100,"Start");
// make path..
line(100,100,500,100);
line(500,100,500,400);
line(500,400,100,400);
line(100,400,100,150);
line(100,150,450,150);
line(450,150,450,350);
line(450,350,150,350);
line(150,350,150,200);
line(150,200,400,200);
line(400,200,400,300);
line(400,300,200,300);
line(200,300,200,250);
line(200,250,350,250);
// starting time
st=clock();
//conditions to out
while(!((x>=100 &&x<=500 && y==100)|| (x==500 && y>=100 && y<=400) ||(x==100 &&
y>=150 && y<=400) ||(x>=100 && x<=500 && y==400) ||(x>=100 && x<=450 && y==150)||(x==450 && y>=150 &&y<=350)||(x>=150 && x<=450 && y==350)||(x==150 &&
y<=350 && y>=200)||(x>=150 && x<=400 && y==200)||(x==400 && y>=200 &&
y<=300)||(x>=200 &&x<=400 && y==300)||(x==200 && y>=250 && y<=300)||(x>=200 &&
x<=350 && y==250) ||(x>200 && x<250 && y>250&& y<300)))
{
key=getch();
setcolor(BLACK);
circle(x,y,6);
if(key=='A' || key=='a')//move up
y--;
else if(key=='Z'||key=='z')//move right
x++;
else if(key=='.')//for downwar
y++;
else if(key=='L' || key=='l')//for left
x--;
setcolor(WHITE);
circle(x,y,6);
//delay(10);
}
// condition for winning
if(x>200 && x<250 && y>250&& y<300)
outtextxy(100,450,"YOU WON");
else
outtextxy(100,450,"YOU LOSE");
char ex;
exee:
ex= getch();
if(ex==13)
ccleardevice();
else goto exee;
//total time taken
cout<<"\n\n\n\tYOU TOOK "<<(clock()-st)/1000<<" Seconds to Play \n\n\n"<<endl;
return 0;
}
Output
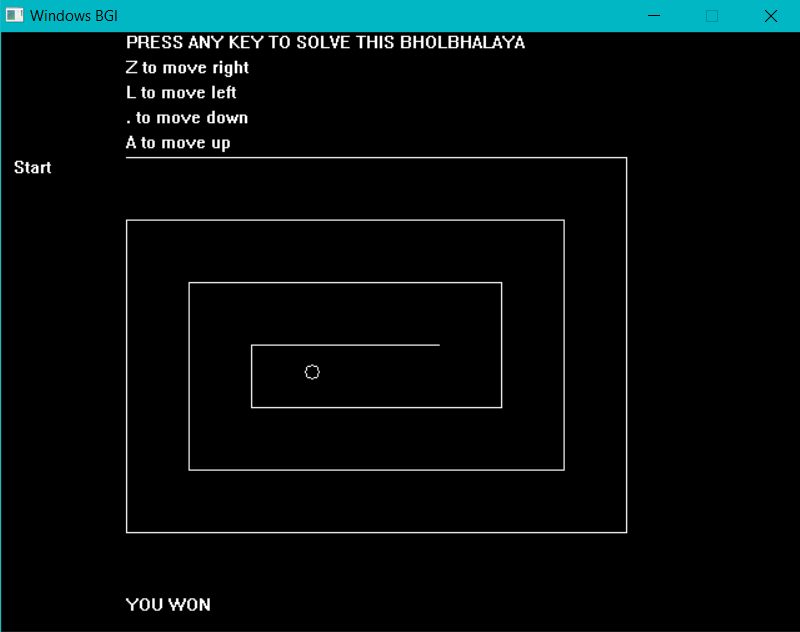